Edit Text is a user input control where user can input something as string or any other data.Edit Text accept any type of data just drag and drop in XML but you have to setInputType to get in specific format data.
User Can get Text Value from Edit Text it can be in simple format or any specific format form above if any.
Simple way to get Text value from Edit Text when user click on Button.
Here I will show some of the validation which we have to check manually while we are getting value form Edit Text.
PersonName :
Here we start with Person Name by default if you drag and set InputType="textPersonName" it will not accept on name or alphabets that also accept numbers . so here i write a code for that only accept alphabets so now when you enter any text then that will check is it text or other symbol ?
Here i wrote a function which we will use in
Now call above function into
Email Address :
InputType="phone" when you enter number it accepted but not in Phone Number Format like if you Enter your Number 9898998989 . It will show same as but if you want to input in format then you have to call that on addTextChangedListener(new PhoneNumberFormattingTextWatcher()); So .its shows like 989-899-8989.
Sign Number :
InputType="numberSigned" i check with minimum and maximum length are valid or not.? call below function in
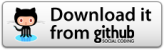
User Can get Text Value from Edit Text it can be in simple format or any specific format form above if any.
Simple way to get Text value from Edit Text when user click on Button.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
| public class EditText_Example extends Activity { protected void onCreate(Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.main); final EditText edt = (Button) findViewById(R.id.EditText_Id); button.setOnClickListener( new View.OnClickListener() { public void onClick(View v) { edt.getText().toStrign(); } }); } } |
public class EditText_Example extends Activity { protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); final EditText edt = (Button) findViewById(R.id.EditText_Id); button.setOnClickListener(new View.OnClickListener() { public void onClick(View v) { edt.getText().toStrign(); } }); } }
Here I will show some of the validation which we have to check manually while we are getting value form Edit Text.
- PersonName
- Email Address
- Phone
- Sign Number
PersonName :
Here we start with Person Name by default if you drag and set InputType="textPersonName" it will not accept on name or alphabets that also accept numbers . so here i write a code for that only accept alphabets so now when you enter any text then that will check is it text or other symbol ?
Here i wrote a function which we will use in
addTextChangedListener
for validating valuw when user are typing the value.
01
02
03
04
05
06
07
08
09
10
11
12
| public void Is_Valid_Person_Name(EditText edt) throws NumberFormatException { if (edt.getText().toString().length() <= 0 ) { edt.setError( "Accept Alphabets Only." ); valid_name = null ; } else if (!edt.getText().toString().matches( "[a-zA-Z ]+" )) { edt.setError( "Accept Alphabets Only." ); valid_name = null ; } else { valid_name = edt.getText().toString(); } } |
public void Is_Valid_Person_Name(EditText edt) throws NumberFormatException { if (edt.getText().toString().length() <= 0) { edt.setError("Accept Alphabets Only."); valid_name = null; } else if (!edt.getText().toString().matches("[a-zA-Z ]+")) { edt.setError("Accept Alphabets Only."); valid_name = null; } else { valid_name = edt.getText().toString(); } }
Now call above function into
addTextChangedListener
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
| name.addTextChangedListener( new TextWatcher() { @Override public void onTextChanged(CharSequence s, int start, int before, int count) { // TODO Auto-generated method stub } @Override public void beforeTextChanged(CharSequence s, int start, int count, int after) { // TODO Auto-generated method stub } @Override public void afterTextChanged(Editable s) { // TODO Auto-generated method stub Is_Valid_Person_Name(name); // pass your EditText Obj here. } }); |
name.addTextChangedListener(new TextWatcher() { @Override public void onTextChanged(CharSequence s, int start, int before, int count) { // TODO Auto-generated method stub } @Override public void beforeTextChanged(CharSequence s, int start, int count, int after) { // TODO Auto-generated method stub } @Override public void afterTextChanged(Editable s) { // TODO Auto-generated method stub Is_Valid_Person_Name(name); // pass your EditText Obj here. } });
Email Address :
InputType="phone" when you enter number it accepted but not in Phone Number Format like if you Enter your Number 9898998989 . It will show same as but if you want to input in format then you have to call that on addTextChangedListener(new PhoneNumberFormattingTextWatcher()); So .its shows like 989-899-8989.
1
| phone_number.addTextChangedListener( new PhoneNumberFormattingTextWatcher()); |
phone_number.addTextChangedListener(new PhoneNumberFormattingTextWatcher());
Sign Number :
InputType="numberSigned" i check with minimum and maximum length are valid or not.? call below function in
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
| public void Is_Valid_Sign_Number_Validation( int MinLen, int MaxLen, EditText edt) throws NumberFormatException { if (edt.getText().toString().length() <= 0 ) { edt.setError( "Sign Number Only" ); valid_sign_number = null ; } else if (Double.valueOf(edt.getText().toString()) < MinLen || Double.valueOf(edt.getText().length()) > MaxLen) { edt.setError( "Out of Range " + MinLen + " or " + MaxLen); valid_sign_number = null ; } else { valid_sign_number = edt.getText().toString(); // Toast.makeText(getApplicationContext(), // ""+edt.getText().toString(), Toast.LENGTH_LONG).show(); } } // END OF Edittext validation |
public void Is_Valid_Sign_Number_Validation(int MinLen, int MaxLen, EditText edt) throws NumberFormatException { if (edt.getText().toString().length() <= 0) { edt.setError("Sign Number Only"); valid_sign_number = null; } else if (Double.valueOf(edt.getText().toString()) < MinLen || Double.valueOf(edt.getText().length()) > MaxLen) { edt.setError("Out of Range " + MinLen + " or " + MaxLen); valid_sign_number = null; } else { valid_sign_number = edt.getText().toString(); // Toast.makeText(getApplicationContext(), // ""+edt.getText().toString(), Toast.LENGTH_LONG).show(); } } // END OF Edittext validation
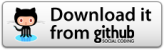
Thanks for the code..easier to use..
ReplyDeleteIt’s great to read content from a writer that cares about quality content. You are intelligent and well-versed on this topic. This is good writing.
ReplyDeleten what abt time
ReplyDeleteFor the date and time you can use Date Picker in android.
ReplyDeletecan you explain me timepicker
ReplyDeleteenth mairile websitanith
ReplyDelete[…] EditText validaton […]
ReplyDeleteEverything is working ..But once user input wrong "Person Name" then Error window appear for permanently...HOw can we dissmiss that error message once user pres back space to correct their name...Pleas Send Solution ASAP..Thanks In Advance
ReplyDeleteInputType=”phone” when you enter number it accepted but not in Phone Number Format like if you Enter your Number 9898998989 . It will show same as but if you want to input in format then you have to call that on addTextChangedListener(new PhoneNumberFormattingTextWatcher()); So .its shows like 989-899-8989.
ReplyDeleteChintan 98989-98989 number is mine kindly remove it from your blog...
thank u :D
ReplyDeletepersone name is not space allowed plz check perfectly
ReplyDeletefor alphanumeric what method we have to use
ReplyDeletecan u please help me out i want to work on native drawer and i am a beginer in android development
ReplyDelete