Hello Guys,
I am here after long time and today i am posting one new sample.
Today I have build simple example in which you can select either Camera or Gallery to pick up an image.For that you have to simple click on Default image and that will launch dialog to ask selection alternative Camera / Gallery.
You can choose any one of them and pick up single image form respective item index either it could be Camera or Gallery.
I will upload next sample in which you can select multiple images from Gallery and use that path.
One Major thing that i have use here and that is
Copy Image to your own created Folder. It means when you Capture any image from Camera or Gallery that image will be copied and pasted into your specific /DIR and we will use that image path from target /dir.
So, You can save and safe your images in your own directory.
Now What you will learn from this post ?
- How to Capture image from camera in android ?
- How to Pick up image from Gallery in android ?
- How to launch alert dialog for image selection either Camera & Gallery ?
- How to create folder in SD Card ?
- How to copy image at specific folder in SD Card ?
- How to create Random File name for image ?
- How to set Header & Footer fix view using Linear Layout in android ?
Let start with XML layout. (I will show only major code here)
Main.xml
|
01 02 03 04 05 06 07 08 09 10 11 12 13 | <LinearLayout
android:layout_width= "fill_parent"
android:layout_height= "fill_parent"
android:layout_weight= "1"
android:gravity= "center"
android:orientation= "vertical" >
<ImageView
android:id= "@+id/user_photo"
android:layout_width= "fill_parent"
android:layout_height= "fill_parent"
android:src= "@drawable/user_logo" />
</LinearLayout>
|
|
|
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:layout_weight="1"
android:gravity="center"
android:orientation="vertical" >
<ImageView
android:id="@+id/user_photo"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:src="@drawable/user_logo" />
</LinearLayout>
Image Selection Mode Camera or Gallery Dialog
- After the selection of one of the mode you will get either Camera screen or Gallery screen , Here you have to select any single image.
- If you are not selecting any image then result should be blank.
|
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | public void Image_Picker_Dialog() {
AlertDialog.Builder myAlertDialog = new AlertDialog.Builder( this );
myAlertDialog.setTitle( "Pictures Option" );
myAlertDialog.setMessage( "Select Picture Mode" );
myAlertDialog.setPositiveButton( "Gallery" , new DialogInterface.OnClickListener() {
public void onClick(DialogInterface arg0, int arg1) {
Utility.pictureActionIntent = new Intent(Intent.ACTION_GET_CONTENT, null );
Utility.pictureActionIntent.setType( "image/*" );
Utility.pictureActionIntent.putExtra( "return-data" , true );
startActivityForResult(Utility.pictureActionIntent, Utility.GALLERY_PICTURE);
}
});
myAlertDialog.setNegativeButton( "Camera" , new DialogInterface.OnClickListener() {
public void onClick(DialogInterface arg0, int arg1) {
Utility.pictureActionIntent = new Intent(android.provider.MediaStore.ACTION_IMAGE_CAPTURE);
startActivityForResult(Utility.pictureActionIntent, Utility.CAMERA_PICTURE);
}
});
myAlertDialog.show();
}
|
|
|
public void Image_Picker_Dialog() {
AlertDialog.Builder myAlertDialog = new AlertDialog.Builder(this);
myAlertDialog.setTitle("Pictures Option");
myAlertDialog.setMessage("Select Picture Mode");
myAlertDialog.setPositiveButton("Gallery", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface arg0, int arg1) {
Utility.pictureActionIntent = new Intent(Intent.ACTION_GET_CONTENT, null);
Utility.pictureActionIntent.setType("image/*");
Utility.pictureActionIntent.putExtra("return-data", true);
startActivityForResult(Utility.pictureActionIntent, Utility.GALLERY_PICTURE);
}
});
myAlertDialog.setNegativeButton("Camera", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface arg0, int arg1) {
Utility.pictureActionIntent = new Intent(android.provider.MediaStore.ACTION_IMAGE_CAPTURE);
startActivityForResult(Utility.pictureActionIntent, Utility.CAMERA_PICTURE);
}
});
myAlertDialog.show();
}
After Selection of Image
- Yeah, Now you have select any single image and that will come with
onActivityResult
.
- Now do some copy & decoding task
|
01 02 03 04 05 06 07 08 09 10 11 | protected void onActivityResult( int requestCode, int resultCode, Intent data) {
super .onActivityResult(requestCode, resultCode, data);
if (requestCode == Utility.GALLERY_PICTURE) {
Image_Selecting_Task(data);
} else if (requestCode == Utility.CAMERA_PICTURE) {
Image_Selecting_Task(data);
}
}
|
|
|
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == Utility.GALLERY_PICTURE) {
// data contains result
// Do some task
Image_Selecting_Task(data);
} else if (requestCode == Utility.CAMERA_PICTURE) {
// Do some task
Image_Selecting_Task(data);
}
}
Copy, Decoding & Bitmap Creation Task
|
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 | public void Image_Selecting_Task(Intent data) {
try {
Utility.uri = data.getData();
if (Utility.uri != null ) {
Cursor cursor = getContentResolver().query(Utility.uri, new String[] {
android.provider.MediaStore.Images.ImageColumns.DATA
}, null , null , null );
cursor.moveToFirst();
final String imageFilePath = cursor.getString( 0 );
Utility.Default_DIR = new File(imageFilePath);
Utility.Create_MY_IMAGES_DIR();
Utility.copyFile(Utility.Default_DIR, Utility.MY_IMG_DIR);
Bitmap b = Utility.decodeFile(Utility.Paste_Target_Location);
String valid_photo = Utility.Paste_Target_Location.toString();
b = Bitmap.createScaledBitmap(b, 150 , 150 , true );
user_photo.setImageBitmap(b);
cursor.close();
} else {
Toast toast = Toast.makeText( this , "Sorry!!! You haven't selecet any image." , Toast.LENGTH_LONG);
toast.show();
}
} catch (Exception e) {
Log.e( "onActivityResult" , "" + e);
}
}
|
|
|
public void Image_Selecting_Task(Intent data) {
try {
Utility.uri = data.getData();
if (Utility.uri != null) {
// User had pick an image.
Cursor cursor = getContentResolver().query(Utility.uri, new String[] {
android.provider.MediaStore.Images.ImageColumns.DATA
}, null, null, null);
cursor.moveToFirst();
// Link to the image
final String imageFilePath = cursor.getString(0);
//Assign string path to File
Utility.Default_DIR = new File(imageFilePath);
// Create new dir MY_IMAGES_DIR if not created and copy image into that dir and store that image path in valid_photo
Utility.Create_MY_IMAGES_DIR();
// Copy your image
Utility.copyFile(Utility.Default_DIR, Utility.MY_IMG_DIR);
// Get new image path and decode it
Bitmap b = Utility.decodeFile(Utility.Paste_Target_Location);
// use new copied path and use anywhere
String valid_photo = Utility.Paste_Target_Location.toString();
b = Bitmap.createScaledBitmap(b, 150, 150, true);
//set your selected image in image view
user_photo.setImageBitmap(b);
cursor.close();
} else {
Toast toast = Toast.makeText(this, "Sorry!!! You haven't selecet any image.", Toast.LENGTH_LONG);
toast.show();
}
} catch (Exception e) {
// you get this when you will not select any single image
Log.e("onActivityResult", "" + e);
}
}
Create Directory if not Exist
|
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | public static File Create_MY_IMAGES_DIR() {
try {
MY_IMG_DIR = new File(Environment.getExternalStorageDirectory(), "/My_Image/" );
if (!MY_IMG_DIR.exists()) {
MY_IMG_DIR.mkdirs();
Log.i( "path" , ">>.." + MY_IMG_DIR);
}
} catch (Exception e) {
Log.e( "Create_MY_IMAGES_DIR" , "" + e);
}
return MY_IMG_DIR;
}
|
|
|
// Create New Dir (folder) if not exist
public static File Create_MY_IMAGES_DIR() {
try {
// Get SD Card path & your folder name
MY_IMG_DIR = new File(Environment.getExternalStorageDirectory(), "/My_Image/");
// check if exist
if (!MY_IMG_DIR.exists()) {
// Create New folder
MY_IMG_DIR.mkdirs();
Log.i("path", ">>.." + MY_IMG_DIR);
}
} catch (Exception e) {
// TODO: handle exception
Log.e("Create_MY_IMAGES_DIR", "" + e);
}
return MY_IMG_DIR;
}
Copy Image at specific Directory in sdcard
|
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | public static File copyFile(File current_location, File destination_location) {
Copy_sourceLocation = new File( "" + current_location);
Paste_Target_Location = new File( "" + destination_location + "/" + Utility.Get_Random_File_Name() + ".jpg" );
Log.v( "Purchase-File" , "sourceLocation: " + Copy_sourceLocation);
Log.v( "Purchase-File" , "targetLocation: " + Paste_Target_Location);
try {
int actionChoice = 2 ;
if (actionChoice == 1 ) {
if (Copy_sourceLocation.renameTo(Paste_Target_Location)) {
Log.i( "Purchase-File" , "Move file successful." );
} else {
Log.i( "Purchase-File" , "Move file failed." );
}
}
else {
if (Copy_sourceLocation.exists()) {
InputStream in = new FileInputStream(Copy_sourceLocation);
OutputStream out = new FileOutputStream(Paste_Target_Location);
byte [] buf = new byte [ 1024 ];
int len;
while ((len = in .read(buf)) > 0 ) {
out.write(buf, 0 , len);
} in .close();
out.close();
Log.i( "copyFile" , "Copy file successful." );
} else {
Log.i( "copyFile" , "Copy file failed. Source file missing." );
}
}
} catch (NullPointerException e) {
Log.i( "copyFile" , "" + e);
} catch (Exception e) {
Log.i( "copyFile" , "" + e);
}
return Paste_Target_Location;
}
|
|
|
// Copy your image into specific folder
public static File copyFile(File current_location, File destination_location) {
Copy_sourceLocation = new File("" + current_location);
Paste_Target_Location = new File("" + destination_location + "/" + Utility.Get_Random_File_Name() + ".jpg");
Log.v("Purchase-File", "sourceLocation: " + Copy_sourceLocation);
Log.v("Purchase-File", "targetLocation: " + Paste_Target_Location);
try {
// 1 = move the file, 2 = copy the file
int actionChoice = 2;
// moving the file to another directory
if (actionChoice == 1) {
if (Copy_sourceLocation.renameTo(Paste_Target_Location)) {
Log.i("Purchase-File", "Move file successful.");
} else {
Log.i("Purchase-File", "Move file failed.");
}
}
// we will copy the file
else {
// make sure the target file exists
if (Copy_sourceLocation.exists()) {
InputStream in = new FileInputStream(Copy_sourceLocation);
OutputStream out = new FileOutputStream(Paste_Target_Location);
// Copy the bits from instream to outstream
byte[] buf = new byte[1024];
int len;
while ((len = in .read(buf)) > 0) {
out.write(buf, 0, len);
} in .close();
out.close();
Log.i("copyFile", "Copy file successful.");
} else {
Log.i("copyFile", "Copy file failed. Source file missing.");
}
}
} catch (NullPointerException e) {
Log.i("copyFile", "" + e);
} catch (Exception e) {
Log.i("copyFile", "" + e);
}
return Paste_Target_Location;
}
[caption id="attachment_117" align="aligncenter" width="164"]
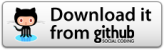
GitHub-download[/caption]