Hello developers, again i am come up with new sample example about camera preview in your XML layout instead of camera activity.
To see camera preview we require SurfaceView and SurfaceHolder.
I have create simple XML Layout file in which i have set two buttons and to show real camera preview we need SurfaceView .
How to mange SurfaceView in java file ?
We will initialize Camera, SurfaceView & SurfaceHolder as global to mange the view. Here is the whole java file which help you easily.
Implement SurfaceHolder.Callback
This will @Override method
Task of Show_Preview & Stop_Preview ?
This is not two different function but i have just filter it so easy to understand.
And don't forget to add
[caption id="attachment_117" align="aligncenter" width="164"]
GitHub-download[/caption]
To see camera preview we require SurfaceView and SurfaceHolder.
I have create simple XML Layout file in which i have set two buttons and to show real camera preview we need SurfaceView .
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | <?xml version= "1.0" encoding= "utf-8" ?> <LinearLayout xmlns:android= "http://schemas.android.com/apk/res/android" android:layout_width= "fill_parent" android:layout_height= "fill_parent" android:orientation= "vertical" > <TextView android:layout_width= "fill_parent" android:layout_height= "wrap_content" android:text= "Hello" /> <Button android:id= "@+id/startcamerapreview" android:layout_width= "fill_parent" android:layout_height= "wrap_content" android:text= "- Start Camera Preview -" /> <Button android:id= "@+id/stopcamerapreview" android:layout_width= "fill_parent" android:layout_height= "wrap_content" android:text= "- Stop Camera Preview -" /> <SurfaceView android:id= "@+id/surfaceview" android:layout_width= "fill_parent" android:layout_height= "wrap_content" /> </LinearLayout> |
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Hello" /> <Button android:id="@+id/startcamerapreview" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="- Start Camera Preview -" /> <Button android:id="@+id/stopcamerapreview" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="- Stop Camera Preview -" /> <SurfaceView android:id="@+id/surfaceview" android:layout_width="fill_parent" android:layout_height="wrap_content" /> </LinearLayout>
How to mange SurfaceView in java file ?
We will initialize Camera, SurfaceView & SurfaceHolder as global to mange the view. Here is the whole java file which help you easily.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 | @Override public void onCreate(Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.main); buttonStartCameraPreview = (Button) findViewById(R.id.startcamerapreview); buttonStopCameraPreview = (Button) findViewById(R.id.stopcamerapreview); surfaceView = (SurfaceView) findViewById(R.id.surfaceview); surfaceHolder = surfaceView.getHolder(); surfaceHolder.addCallback( this ); // write below code inside this block surfaceHolder.setType(SurfaceHolder.SURFACE_TYPE_PUSH_BUFFERS); buttonStartCameraPreview.setOnClickListener(); // write below code inside this block buttonStopCameraPreview.setOnClickListener(); } |
@Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); buttonStartCameraPreview = (Button) findViewById(R.id.startcamerapreview); buttonStopCameraPreview = (Button) findViewById(R.id.stopcamerapreview); surfaceView = (SurfaceView) findViewById(R.id.surfaceview); surfaceHolder = surfaceView.getHolder(); surfaceHolder.addCallback(this); // write below code inside this block surfaceHolder.setType(SurfaceHolder.SURFACE_TYPE_PUSH_BUFFERS); buttonStartCameraPreview.setOnClickListener(); // write below code inside this block buttonStopCameraPreview.setOnClickListener(); }
Implement SurfaceHolder.Callback
This will @Override method
surfaceChanged , surfaceCreated , surfaceDestroyed
You can write your optional code here as per your requirement.Task of Show_Preview & Stop_Preview ?
This is not two different function but i have just filter it so easy to understand.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | Show_Preview() { if (!previewing) { camera = Camera.open(); if (camera != null ) { try { camera.setPreviewDisplay(surfaceHolder); camera.startPreview(); previewing = true ; } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } } Stop_Preview() { if (camera != null && previewing) { camera.stopPreview(); camera.release(); camera = null ; previewing = false ; } } @Override public void onClick(View v) { // TODO Auto-generated method stub if (v.getId() == R.id.startcamerapreview) { Show_Preview(); } if (v.getId() == R.id.stopcamerapreview) { Stop_Preview(); } } |
Show_Preview() { if (!previewing) { camera = Camera.open(); if (camera != null) { try { camera.setPreviewDisplay(surfaceHolder); camera.startPreview(); previewing = true; } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } } Stop_Preview() { if (camera != null && previewing) { camera.stopPreview(); camera.release(); camera = null; previewing = false; } } @Override public void onClick(View v) { // TODO Auto-generated method stub if (v.getId() == R.id.startcamerapreview) { Show_Preview(); } if (v.getId() == R.id.stopcamerapreview) { Stop_Preview(); } }
And don't forget to add
android:name="android.permission.CAMERA"
in Manifest file.[caption id="attachment_117" align="aligncenter" width="164"]
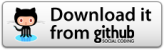
your samples are very nice.. can share some sample examples on Conten Provider.
ReplyDeleteHey Chintan great tutorial bro !
ReplyDeleteJust wanted to say that the camera is somewhat tilted when I run the application on my device.
Can you say what possibly could be the problem ?
Do you mean there is an action bar? You can hide it or disable it. Easier way is to run
ReplyDeletegetActionBar().hide() in your onCreate(Bundle s) of your activity.
hi,
ReplyDeletethere is camera issue that rotates in potrate
It's device specific bug you need to rotate manually.
ReplyDeletehi, can we have a camera preview of front facing camera and back side by side .if it is so can u tell me how
ReplyDelete